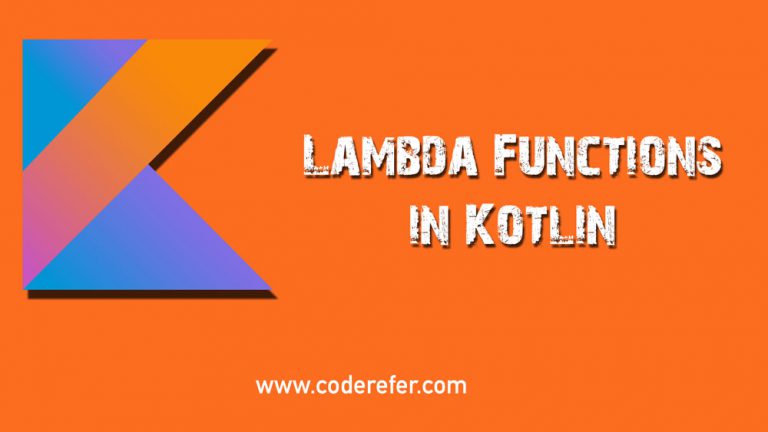
Lambda function is powerful feature in any Programming language and Lambda function in Kotlin is no exception. In this article, we will look at how to create Lambda function in Kotlin along with simple examples.
By the end of this article, you will be able to learn:
- What is a Lambda Function
- How to Create a Lambda function in Kotlin
- Practical Examples where Lambda Function are used
You may also like:
Higher Order Functions in Kotlin
Let us quickly start on what is a Lambda function.
What is a Lambda Function?
A Lambda Function is an Anonymous function which we can use similar to variables, i.e., we can either pass them as arguments to Functions or we can return the lambdas.
The functions which accepts Lambdas are called Higher order functions, which we have discussed in our previous article. If you haven’t already gone through, I would highly recommend going through this article.
How to Create a Lambda Function in Kotlin
Before creating a Lambda function, let us first see its syntax example.
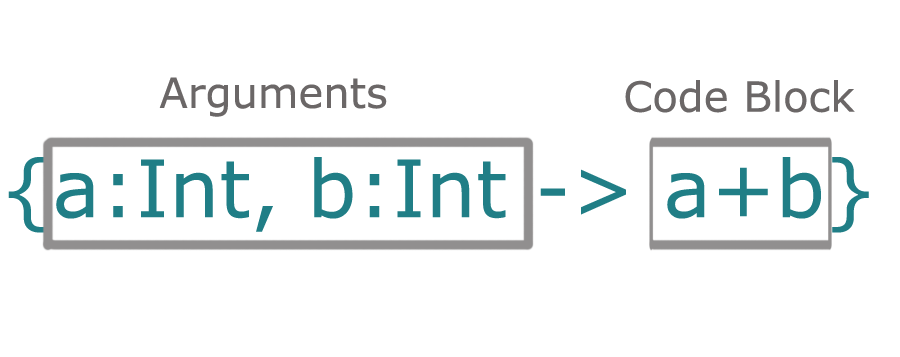
In the above image, we can see that in left side of arrow, arguments are written and in right side, function block is declared. Curly braces are mandatory to write a lambda function in Kotlin.
Now to learn how to write a Lambda function, let us take a normal function in Kotin and there after modify it.
Here is a typical normal kotlin Function
// Normal function | |
fun area(length:Int, breadth: Int) : Int{ | |
return length * breadth | |
} |
Now, if we convert the same function into a Lambda, the result would be:
{ length: Int, breadth: Int -> (length * breadth) } |
We can clearly see that using a lambda turned the above function into a single liner.
Let us now declare the above function as a Lambda Expression
//Lambda Expression | |
val areaOfRectangle = { length: Int, breadth: Int -> (length * breadth) } |
In the above gist, we declared a lambda function and assigned it to a variable. Now we can call the above lambda expression and pass the arguments as shown below:
fun main() { | |
areaOfRectangle(10,20) | |
} |
We can also declare the lambda expression as shown below which is slightly different from the above example.
//simplified version | |
val areaOfRectangleSimplified: (Int, Int) -> Int = { length, breadth -> length * breadth } |
From the above gist, we can see that we have simplified the right part by removing the data types declared. The type of those lambda’s arguments are inferred from the variable type which we have declared in the left part.
This simplified expression can be called similar to the above example as shown in below
fun main() { | |
areaOfRectangleSimplified(30,40) | |
} |
Now let us see few practical examples of how a Lambda function in Kotlin is used in Higher Order Functions.
Practical Examples of Lambda Function in Kotlin
Let us create a list and use forEach() function available in Kotlin’s Collection class.
val list = listOf(1, 3, 5, 7, 9) | |
// a function taking a single function as a parameter | |
list.forEach({ println(it) }) //it -> iterator which iterates and gives each value of list |
forEach() function accepts a Lambda as an Argument which we have used to print each of the list item in line 3. Here “it” refers to iterator which gives us each value of list item in each iteration.
In Kotlin, we can omit parenthesis if a function has only a single lambda argument.
// if parameter is a single lambda expression, we can omit the paranthesis | |
list.forEach { println() } |
Now let’s use the fold() function available in Kotlin Collection class.
val list = listOf(1, 3, 5, 7, 9) | |
// fold function is taking a parameter and a lambda expression | |
println(list.fold(1, { a, b -> a * b })) |
Here in fold function, the second argument accepts Lambda Function. The output of the above is 945.
In Kotlin, if the function’s last argument is a Lambda Function, we can write the Lambda Function outside of function. Hence the above function can be written as follows:
val list = listOf(1, 3, 5, 7, 9) | |
// if the lambda expression is the last parameter, we can move the parameter out | |
println(list.fold(1) { a, b -> a * b }) |
The output of the above is also same, i.e., 945.
With this examples, I would be concluding this article. In the next article, let us see closures in Kotlin and their comparision with Java. Comment below to let us know the feedback / queries.
Reference article:Â kotlinlang